Mesh functions¶
Expressions can be used to access functions available in Mesh. The result of a calculation based on a function is a temporary time series, i.e. a time series which is not in the database. Every time the calculation expression is run, values are calculated for the temporary time series. However, the result is only available when the Mesh session is open.
The Mesh search language can be used with functions to find specific objects to work on.
Definition of a Mesh function¶
Functions are identified with a name and an unprotected identifier, and are distinguished from variables by the first character: an @-symbol. The same function name can have several argument combinations.
The function’s argument is placed in parenthesis ( ). A function can have any number of arguments, including 0.
Example showing expressions made using functions:
- Expression A equals a number
A = 5
- Expression B equals the result of the sum of two MAX functions using expression A and the max value in time series EnTS
B = @MAX(A, 55) + @MAX(%EnTS)
Valid result types from functions:
Single time series
Array of time series
Single double value
Array of double values
Single string value
Array of string values
Forecast¶
A forecast is a calculation or estimate of future events, especially coming weather or a financial trend.
Every grey line in the image below represents a forecast of some time series. Start and end times are given on the horizontal axis, while the vertical axis shows every forecasts write time.
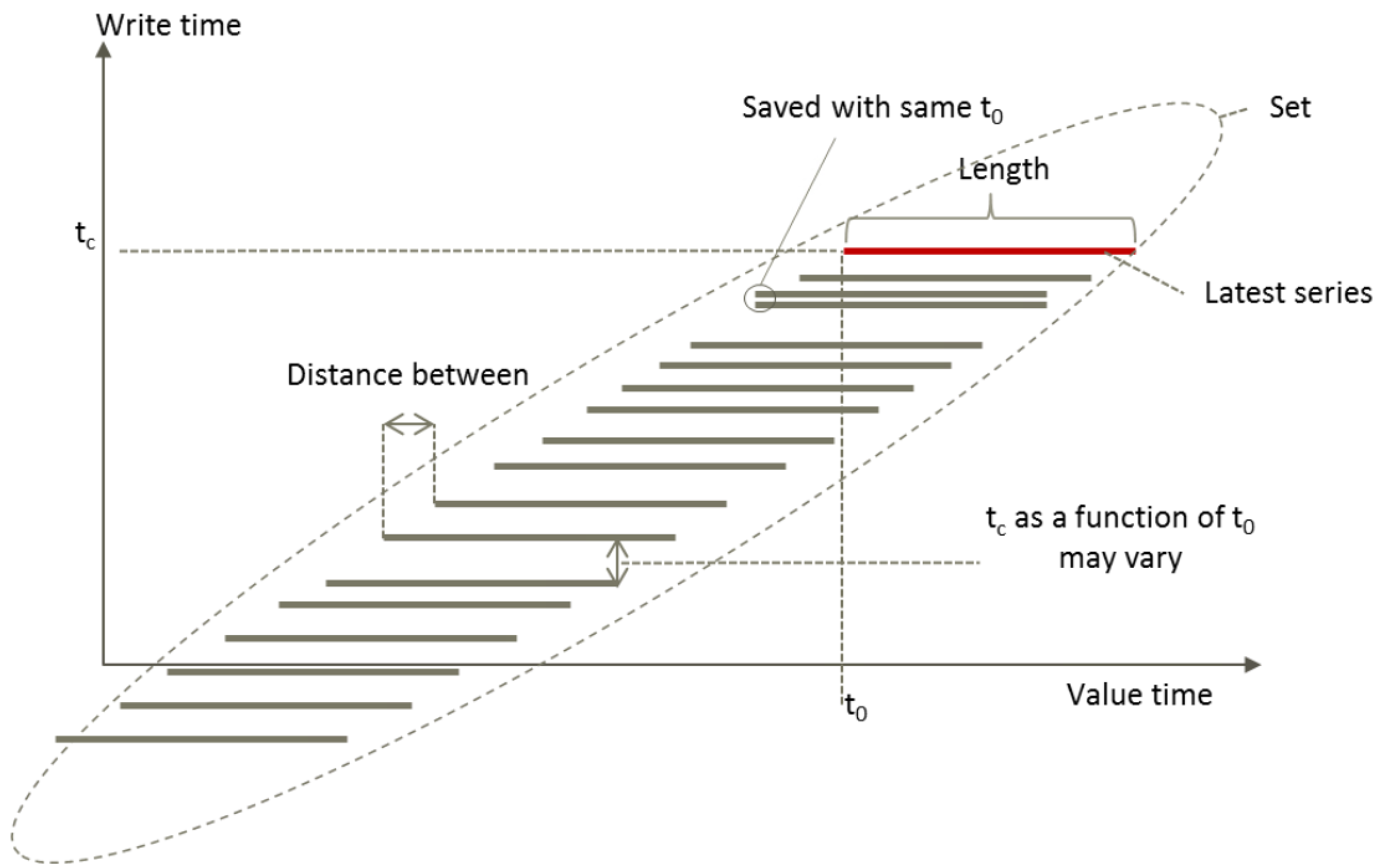
- class volue.mesh.calc.forecast.ForecastFunctions(session, target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime)[source]
Class for forecast functions that should be run synchronously
- get_all_forecasts(search_query: Optional[str] = None) List[Timeseries] [source]
Get all forecasts for a given Mesh object in a time interval. The target and the time interval (start_time and end_time) are set by
volue.mesh.Connection.Session.forecast_functions()
.Example
If interval ‘P’ is given for the Mesh object in the picture below, 10 forecasted time series will be returned.
Note
The resulting objects from the search_query will be used in the get_all_forecasts function, if search_query is not set the target will be used.
- Parameters
search_query – A search formulated using the Mesh search language.
- Returns
An array of forecast time series with values within the relevant period. Values in forecast series outside the period are not included. The function returns an empty array if no forecast time series have values within the relevant period.
- get_forecast(forecast_start_min: Optional[datetime] = None, forecast_start_max: Optional[datetime] = None, available_at_timepoint: Optional[datetime] = None, search_query: Optional[str] = None) Timeseries [source]
Get one forecast for a given Mesh object in a time interval.
The target`and the time interval (`start_time and end_time) are set by
volue.mesh.Connection.Session.forecast_functions()
.- Example 1:
Use available_at_timepoint (tc) to get the forecast.
forecast_funcs = session.forecast_functions(full_name, start_time, end_time) result = forecast_funcs.get_forecast(available_at_timepoint)
- Example 2:
Use forecast_start_min (t0min) and forecast_start_max (t0max) to get the forecast that starts in that interval.
Note: This will ignore start_time set by
volue.mesh.Connection.Session.forecast_functions()
forecast_funcs = session.forecast_functions(full_name, start_time, end_time) result = forecast_funcs.get_forecast(forecast_start_min, forecast_start_max)
Note
The function can take available_at_timepoint without specifying forecast_start_min and forecast_start_min.
The function can take forecast_start_min and forecast_start_min with or without specifying available_at_timepoint to find the relevant forecast instead of using the start of the requested period (defined in forecast_functions). It requires that the forecast series’ start is less than or equal to forecast_start_max and larger than forecast_start_min.
If no forecast series has its start time within the given interval, the function returns a time series with NaN.
The resulting objects from the search_query will be used in the get_all_forecasts function, if search_query is not set the target will be used.
- Parameters
forecast_start_min – Forecast must start after this time.
forecast_start_max – Forecast must start before this time.
available_at_timepoint – Forecast that is valid at the given timestamp.
search_query – A search formulated using the Mesh search language.
See also
- Returns
A time series forecast.
- class volue.mesh.calc.forecast.ForecastFunctionsAsync(session, target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime)[source]
Class for forecast functions that should be run asynchronously
- async get_all_forecasts(search_query: Optional[str] = None) List[Timeseries] [source]
Get all forecasts for a given Mesh object in a time interval. The target and the time interval (start_time and end_time) are set by
volue.mesh.Connection.Session.forecast_functions()
.Example
If interval ‘P’ is given for the Mesh object in the picture below, 10 forecasted time series will be returned.
Note
The resulting objects from the search_query will be used in the get_all_forecasts function, if search_query is not set the target will be used.
- Parameters
search_query – A search formulated using the Mesh search language.
- Returns
An array of forecast time series with values within the relevant period. Values in forecast series outside the period are not included. The function returns an empty array if no forecast time series have values within the relevant period.
- async get_forecast(forecast_start_min: Optional[datetime] = None, forecast_start_max: Optional[datetime] = None, available_at_timepoint: Optional[datetime] = None, search_query: Optional[str] = None) Timeseries [source]
Get one forecast for a given Mesh object in a time interval.
The target`and the time interval (`start_time and end_time) are set by
volue.mesh.Connection.Session.forecast_functions()
.- Example 1:
Use available_at_timepoint (tc) to get the forecast.
forecast_funcs = session.forecast_functions(full_name, start_time, end_time) result = forecast_funcs.get_forecast(available_at_timepoint)
- Example 2:
Use forecast_start_min (t0min) and forecast_start_max (t0max) to get the forecast that starts in that interval.
Note: This will ignore start_time set by
volue.mesh.Connection.Session.forecast_functions()
forecast_funcs = session.forecast_functions(full_name, start_time, end_time) result = forecast_funcs.get_forecast(forecast_start_min, forecast_start_max)
Note
The function can take available_at_timepoint without specifying forecast_start_min and forecast_start_min.
The function can take forecast_start_min and forecast_start_min with or without specifying available_at_timepoint to find the relevant forecast instead of using the start of the requested period (defined in forecast_functions). It requires that the forecast series’ start is less than or equal to forecast_start_max and larger than forecast_start_min.
If no forecast series has its start time within the given interval, the function returns a time series with NaN.
The resulting objects from the search_query will be used in the get_all_forecasts function, if search_query is not set the target will be used.
- Parameters
forecast_start_min – Forecast must start after this time.
forecast_start_max – Forecast must start before this time.
available_at_timepoint – Forecast that is valid at the given timestamp.
search_query – A search formulated using the Mesh search language.
See also
- Returns
A time series forecast.
The above class is exposed via:
- Session.forecast_functions(target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime) ForecastFunctions
Access to Forecast functions.
- Parameters
target – Mesh object, attribute, virtual or physical time series the calculation expression will be evaluated relative to. It could be a time series key, Universal Unique Identifier or a path in the Mesh model.
start_time – the start date and time of the time series interval
end_time – the end date and time of the time series interval
- Returns
Object containing all forecast functions.
- Session.forecast_functions(target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime) ForecastFunctionsAsync
Access to Forecast functions.
- Parameters
target – Mesh object, attribute, virtual or physical time series the calculation expression will be evaluated relative to. It could be a time series key, Universal Unique Identifier or a path in the Mesh model.
start_time – the start date and time of the time series interval
end_time – the end date and time of the time series interval
- Returns
Object containing all forecast functions.
History¶
Historical values are not overwritten when saving new values. The figure below shows a time series having values with various write times. When asking for historical values before a given time (tc) the values indicated by red are returned.
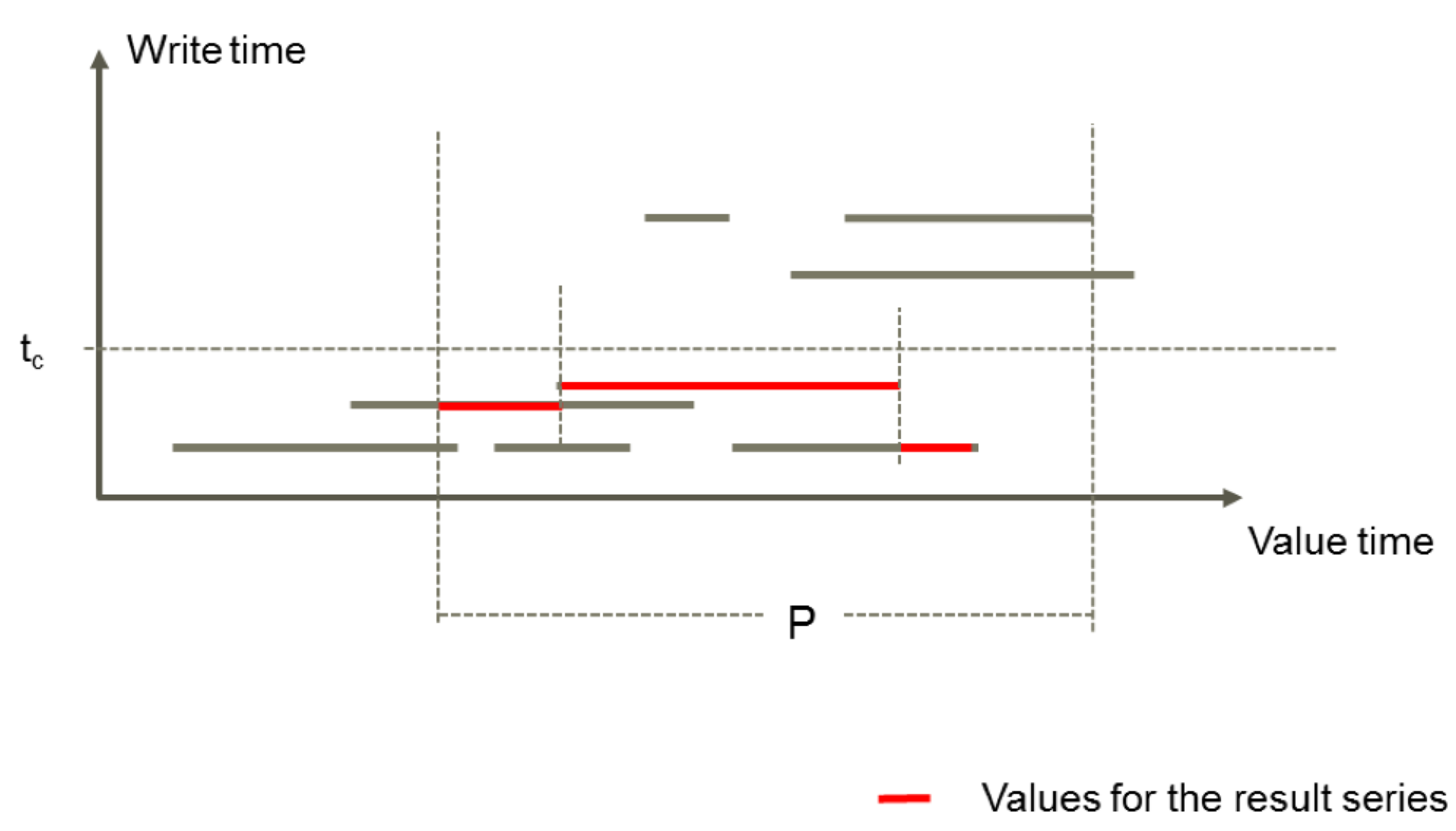
- class volue.mesh.calc.history.HistoryFunctions(session, target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime)[source]
Class for history functions that should be run synchronously
- get_ts_as_of_time(available_at_timepoint: datetime, search_query: Optional[str] = None) Timeseries [source]
Finds values and status for a time series at a given historical time available_at_timepoint.
Note
The resulting objects from the search_query will be used in the get_ts_as_of_time function, if search_query is not set the target will be used.
If the historical time is earlier than the first write to the series (in the relevant period) then the function returns NaN values.
- Parameters
available_at_timepoint – Is valid at the given timestamp.
search_query – A search formulated using the Mesh search language.
For information about datetime arguments and time zones refer to Date times and time zones.
- Returns
A time series with historical values.
- get_ts_historical_versions(max_number_of_versions_to_get: int, search_query: Optional[str] = None) List[Timeseries] [source]
Requests an array of a given number of versions of a time series.
Examples
GetTsHistoricalVersions(ts,1) returns the last change made, i.e. the latest historical version that is different from the current time series.
GetTsHistoricalVersions(ts,3) returns the three last changes. The first series displays the state before the last change, the second displays the state before the second last change, etc.
- Parameters
max_number_of_versions_to_get – Maximum number of time series to return.
search_query – A search formulated using the Mesh search language.
Note
The resulting objects from the search_query will be used in the get_ts_historical_versions function, if search_query is not set the target will be used.
- Returns
An array of time series with historical values.
- class volue.mesh.calc.history.HistoryFunctionsAsync(session, target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime)[source]
Class for history functions that should be run asynchronously
- async get_ts_as_of_time(available_at_timepoint: datetime, search_query: Optional[str] = None) Timeseries [source]
Finds values and status for a time series at a given historical time available_at_timepoint.
Note
The resulting objects from the search_query will be used in the get_ts_as_of_time function, if search_query is not set the target will be used.
If the historical time is earlier than the first write to the series (in the relevant period) then the function returns NaN values.
- Parameters
available_at_timepoint – Is valid at the given timestamp.
search_query – A search formulated using the Mesh search language.
For information about datetime arguments and time zones refer to Date times and time zones.
- Returns
A time series with historical values.
- async get_ts_historical_versions(max_number_of_versions_to_get: int, search_query: Optional[str] = None) List[Timeseries] [source]
Requests an array of a given number of versions of a time series.
Examples
GetTsHistoricalVersions(ts,1) returns the last change made, i.e. the latest historical version that is different from the current time series.
GetTsHistoricalVersions(ts,3) returns the three last changes. The first series displays the state before the last change, the second displays the state before the second last change, etc.
- Parameters
max_number_of_versions_to_get – Maximum number of time series to return.
search_query – A search formulated using the Mesh search language.
Note
The resulting objects from the search_query will be used in the get_ts_historical_versions function, if search_query is not set the target will be used.
- Returns
An array of time series with historical values.
The above class is exposed via:
- Session.history_functions(target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime) HistoryFunctions
Access to History functions.
- Parameters
target – Mesh object, attribute, virtual or physical time series the calculation expression will be evaluated relative to. It could be a time series key, Universal Unique Identifier or a path in the Mesh model.
start_time – the start date and time of the time series interval
end_time – the end date and time of the time series interval
- Returns
Object containing all history functions.
- Session.history_functions(target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime) HistoryFunctionsAsync
Access to History functions.
- Parameters
target – Mesh object, attribute, virtual or physical time series the calculation expression will be evaluated relative to. It could be a time series key, Universal Unique Identifier or a path in the Mesh model.
start_time – the start date and time of the time series interval
end_time – the end date and time of the time series interval
- Returns
Object containing all history functions.
Statistical¶
Functions for performing statistical operations on time series.
Mesh calculation statistical functions.¶
For more information see Statistical.
- class volue.mesh.calc.statistical.StatisticalFunctions(session, target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime)[source]
Class for statistical functions that should be run synchronously
- sum(search_query: Optional[str] = None)[source]
Calculates the sum of all of the series in an array of time series. The resulting time series is equal to the sum of the values for each time interval in the expression.
- Parameters
search_query – A search formulated using the Mesh search language.
Note
The resulting objects from the search_query will be used in the sum function, if search_query is not set the target will be used.
- Returns
A time series with the sum of the values for each time interval in the expression.
- sum_single_timeseries(search_query: Optional[str] = None)[source]
Calculates the sum of the values of the time series for the required period. It returns a number.
- Parameters
search_query – A search formulated using the Mesh search language.
Note
The resulting object (single time series) from the search_query will be used in the sum_single_timeseries function, if search_query is not set the target will be used.
- Returns
The sum of the values of the time series for the required period.
- class volue.mesh.calc.statistical.StatisticalFunctionsAsync(session, target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime)[source]
Class for statistical functions that should be run asynchronously
- async sum(search_query: Optional[str] = None)[source]
Calculates the sum of all of the series in an array of time series. The resulting time series is equal to the sum of the values for each time interval in the expression.
- Parameters
search_query – A search formulated using the Mesh search language.
Note
The resulting objects from the search_query will be used in the sum function, if search_query is not set the target will be used.
- Returns
A time series with the sum of the values for each time interval in the expression.
- async sum_single_timeseries(search_query: Optional[str] = None)[source]
Calculates the sum of the values of the time series for the required period. It returns a number.
- Parameters
search_query – A search formulated using the Mesh search language.
Note
The resulting object (single time series) from the search_query will be used in the sum_single_timeseries function, if search_query is not set the target will be used.
- Returns
The sum of the values of the time series for the required period.
The above class is exposed via:
- Session.statistical_functions(target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime) StatisticalFunctions
Access to Statistical functions.
- Parameters
target – Mesh object, attribute, virtual or physical time series the calculation expression will be evaluated relative to. It could be a time series key, Universal Unique Identifier or a path in the Mesh model.
start_time – the start date and time of the time series interval
end_time – the end date and time of the time series interval
- Returns
Object containing all statistical functions.
- Session.statistical_functions(target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime) StatisticalFunctionsAsync
Access to Statistical functions.
- Parameters
target – Mesh object, attribute, virtual or physical time series the calculation expression will be evaluated relative to. It could be a time series key, Universal Unique Identifier or a path in the Mesh model.
start_time – the start date and time of the time series interval
end_time – the end date and time of the time series interval
- Returns
Object containing all statistical functions.
Transform¶
The functions in this category are used to transform a time series from one resolution to another.
- class volue.mesh.calc.transform.Method(value, names=<not given>, *values, module=None, qualname=None, type=None, start=1, boundary=None)[source]
Methods used for transforming a time series from one resolution to another.
- Parameters
SUM – The sum of the values included in the base for this value. Does not consider how long the values are valid, i.e. a break point series with two values in the current interval that will give the sum of these two values.
SUMI – Integral based sum with resolution second. Calculates the sum of value multiplied with number of seconds each value is valid. Value equal 1 at the start of the day will give 86400 as day value if the base is one break point series and 3600 if this is an hour series with only one value on first hour.
AVG – For fixed interval series. Sum of all values in accumulation period divided by number of values in the accumulation period (24 for hour series that is transformed to day series). For break point series: Mean value of the values included in the base for this value. Does not consider how long the values are valid, i.e. a break point series with two values in the current interval that will give the mean value of these two values.
AVGI – Integral based mean value, i.e. considers how much of the accumulation period that a given value is valid (to next value that can be NaN for a fixed interval series).
FIRST – First value in the accumulation period. For break point series this is the functional value at the start of the accumulation period, unless there exist an explicit value. Please note! For fixed interval series it is the first value not being NaN in the accumulation period.
LAST – Last value in the accumulation period. For break point series this is the functional value at the end of the accumulation period, unless there exist an explicit value. Note! For fixed interval series it is the last value not being NaN in the accumulation period.
MIN – Smallest value in the accumulation period.
MAX – Largest value in the accumulation period.
- AVG = 2
- AVGI = 3
- FIRST = 5
- LAST = 6
- MAX = 8
- MIN = 7
- SUM = 0
- SUMI = 1
- class volue.mesh.calc.transform.TransformFunctions(session, target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime)[source]
Class for transformation functions that should be run synchronously
- transform(resolution: Resolution, method: Method, timezone: Timezone = Timezone.UTC, search_query: Optional[str] = None) Timeseries [source]
Transforms time series from one resolution to another resolution.
Some of target resolutions have a time zone foundation. Note: the LOCAL and STANDARD time zone refers to time zone of Mesh server, not the Python client.
Example
DAY can be related to European Standard Time (UTC+1), which is different from the DAY scope in Finland (UTC+2). When the time zone argument to TRANSFORM is omitted, the configured standard time zone with no Daylight Saving Time enabled is used. You can use it to convert both ways, i.e. both from finer to coarser resolution, and the other way. The most common use is accumulation, i.e. transformation to coarser resolution. Most transformation methods are available for this latter use.
- Parameters
resolution – The resolution to transform to.
method – What method to use for the transformation.
timezone – What time zone to use for the transformation.
search_query – a search formulated using the Mesh search language.
Note
The resulting objects from the search_query will be used in the transform function, if search_query is not set the target will be used.
- Returns
A time series with transformed values.
- class volue.mesh.calc.transform.TransformFunctionsAsync(session, target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime)[source]
Class for transformation functions that should be run asynchronously
- async transform(resolution: Resolution, method: Method, timezone: Timezone = Timezone.UTC, search_query: Optional[str] = None) Timeseries [source]
Transforms time series from one resolution to another resolution.
Some of target resolutions have a time zone foundation. Note: the LOCAL and STANDARD time zone refers to time zone of Mesh server, not the Python client.
Example
DAY can be related to European Standard Time (UTC+1), which is different from the DAY scope in Finland (UTC+2). When the time zone argument to TRANSFORM is omitted, the configured standard time zone with no Daylight Saving Time enabled is used. You can use it to convert both ways, i.e. both from finer to coarser resolution, and the other way. The most common use is accumulation, i.e. transformation to coarser resolution. Most transformation methods are available for this latter use.
- Parameters
resolution – The resolution to transform to.
method – What method to use for the transformation.
timezone – What time zone to use for the transformation.
search_query – a search formulated using the Mesh search language.
Note
The resulting objects from the search_query will be used in the transform function, if search_query is not set the target will be used.
- Returns
A time series with transformed values.
The above class is exposed via:
- Session.transform_functions(target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime) TransformFunctions
Access to Transform functions.
- Parameters
target – Mesh object, attribute, virtual or physical time series the calculation expression will be evaluated relative to. It could be a time series key, Universal Unique Identifier or a path in the Mesh model.
start_time – the start date and time of the time series interval
end_time – the end date and time of the time series interval
- Returns
Object containing all transformation functions.
- Session.transform_functions(target: Union[UUID, str, int, AttributeBase, Object], start_time: datetime, end_time: datetime) TransformFunctionsAsync
Access to Transform functions.
- Parameters
target – Mesh object, attribute, virtual or physical time series the calculation expression will be evaluated relative to. It could be a time series key, Universal Unique Identifier or a path in the Mesh model.
start_time – the start date and time of the time series interval
end_time – the end date and time of the time series interval
- Returns
Object containing all transformation functions.